*About Command Line Utilities*
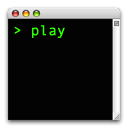
These simple command-line utilities are aimed at facilitating bridging the gap between musicianship and engineering.
The idea is picking the simplest examples for showing how to make "writing" the sound on a computer possible,
without staying on the surface. The "toolbox" includes 25+ simple programs with source code showing "what, how and why".
Why command-line utilities?
-
because they are basic.
-
because they encourage curiosity, freedom of learning and do-it-yourself research "hunch".
-
because they can mitigate a learning curve.
-
because these utilities hadn't been found elsewhere.
-
because they are simple enough to write and require no professional programming skills in order to be written.
Just a bit of plain
C language,
no streamlined shell-scripts, classes and wrappers.
-
because the only audio-specific tools used, if any, are the most basic,
lowest-level CoreAudio or
RTaudio tools.
-
because the documentation on how to write some of them is still surprisingly scarce.
-
because they have proved useful so far.
-
because even the most sophisticated algorithmic composition languages, such as
RTcmix are command-line based.
Here are but a few:

Starters
This is the "Hello, World!" for starting something with sound in C.
Five little programs for producing consecutive raw values of a signal. They cannot play,
but are here to help understanding how basic things work.
USAGE:
table size
sine num_cycles num_steps
sinef num_cycles num_steps destfile
siner duration frequency sampling_rate
ks duration frequency sampling_rate
Please read the README.txt file enclosed.

PartialsCatenator
Beside raw data there are also analytical documents which help musically representing and controlling
the computer as instrument. One such type is the "partial tracking analysis".
This UNIX utility written in plain "Hello, World!"-style C
serves for appending partials
from different analysis file into a single file. They only have to be of the same format,
which is the old-style LISP list, as explained in
SculptView
help file.
USAGE:
ptcat file1 file2 ... ...filen > destfile
If you don't redirect output to a destfile, it will print on the screen.

Partials' Converters
They convert between two prevailing text formats for partial analysis data.
Here are two of them:
-
s2l converts SPEAR format to old-style LISP format.
USAGE:
s2l < infile > outfile
If you don't redirect output to a outfile, it will print on the screen.
-
l2s converts old-style LISP format to newer SPEAR format.
USAGE:
l2s < infile > outfile
If you don't redirect output to a outfile, it will print on the screen.
SDIF text files are way more tricky to deal with, and often involve some rather
sophisticated content interpretation. You should use
SculptView
or SPEAR to do the job.

Partial Tracking File Resampler
This is
utility "resamples" existing patrial tracking file using uniform distance among
breakpoints and cubic spline interpolation. It involves a bit more advanced math, and the
number crunching code is based on the 1988 Columbia University Computer Science Dept.
Technical Report CUCS-389-88 by George Wolberg: CUBIC SPLINE INTERPOLATION: A REVIEW.
USAGE:
ptfr file_name <dur>
- dur is the desired uniform interval duration between points.
Upon resampling completion the program will prompt for the name of a new file to save to.

SineWaveGenerator
This is a CoreAudio
utility serving for ad-hoc sonification of numbers
which represent arbitrary sets of partial frequencies, and for exploring how they relate
to tone timbres. Also for easily demonstratning the concept of additive synthesis.
Numbers are passed as a string of command line arguments, possibly
followed by a - sign and an optional letter for a spectral
envelope:
q for quadratic
e for exponential
b for Bessel J0
Default (no option) is linear reciprocal
USAGE:
swg freq1 freq2 ... ...freqn -[q|e|b]
Frequencies are in c.p.s [=Hz]
Pressing "Enter" starts the playback.
Sine wave sounds of chosen frequencies are being played simultaneously and single
sample-precision phase-accurately.
Pressing "Enter" also stops the playback.

FloatBufferPlayer
Here is the simplest
CoreAudio
utility for playing plain binary files of arbitrary
floating point data, which may not have been "born" as audio
streams, but may have been generated elsewhere, not even as audio files.
Therefore they miss their "id"-s, header, packets,
encodings, audio descriptors, and all the useful, cool and hype stuff which help the format
standardization in entertainment and music industry stay public, but turn even the most humble
attempts of non-disciplinary thinking into acts of banging against a wall of elaborate industry
standards.
USAGE:
fbp file
File has to be binary, with 32-bit floating-point values.
It will get interpreted and buffered as a stream of momentary amplitude values.
It will be played through the default audio output at the default sample rate.
Volume normalizer is part of the utility, so it shouldn't blast your speakers.
Pressing "Enter" starts the playback.
Pressing "Enter" also stops the playback.
The GUI version
of this program can also save the values as CAF and AIF audio files.
TextFilePlayer is a version of the same program
which can read one dimensional floating point data in text format
and converts it to sound; it is available in source code.
USAGE:
tfp file

FloatByteSwapper
This is a Float32 format
byte-swapping
utility, just in case the floating-point data you want to sonify comes in non-native endianness.
USAGE:
fbs file.fp -[option]
option n = normalize
File has to be binary, with 32-bit floating-point values.

Thread Demo
This is a dummy simulator for a
multi-threading
procedure similar to those commonly used for number crunching in digital synthesis. It takes desired data buffer length
from command-line, checks how many CPUs are available, and generates that many threads, each with its
own data buffer. Each thread performs test computation, filling its buffer with numbers until done. On
joining threads, data buffers are being summed and the sums printed.
USAGE:
threads -[option]
option n = no print to disable printing (which takes its CPU toll)
Enter buffer length:
For getting a view on what the program does enter a small number, such as 10.
For monitoring the CPU load enter a higher number, such as 1000000.

Slightly more elaborate examples:
These programs represent a functional upgrade of the programs above. It is possible to connect the programs in the command line interface,
piping output of one program int the input of the other. Source code shows implementation of redirection, event loop and signals.
- algorithmic frequency series generator:
- pri generates frequency series derived from prime numbers inside [n1 n2] interval
mapped into twelve pitch classes, realized in rectus and inversus form.
USAGE: com <n1> <n2>, n1 and n2 are positive integers.
- com generates frequency series from binomial coefficients mapped into twelve
pitch classes, realized in rectus and inversus form.
USAGE: com <n>, n is a positive integer.
- genetic generates series of n frequencies from genetic agorithm results, mapped into twelve
pitch classes.
USAGE: com <n>, n is a positive integer.
- sinewave synthesizer:
- siner1 generates sinewave samples from frequency series, in text or
binary format, for hard-coded sampling frequency (44100Hz) and adjustable segment duration.
USAGE: siner1 [-bd duration], option b stands for binary output format, option d requires segment duration (in seconds).
- players:
- tfp1 accepts text format data
- fbp1 accepts binary format data
- connection examples:
- pri 1 128 | siner1 | tfp1
- com 6 | siner1 -bd 0.2 | fbp1

RtAudio examples
Writing simple, meaningful low-level audio code can some times be a bit of challenge.
These are few basic code examples in C++, using the OS-independent, portable
RtAudio
library and API:
-
rtsine plays a musical interval of two sinewaves in L and R channels.
USAGE:
rtsine
-
rtswg is the C++ source code for the additive SineWaveGenerator
USAGE:
rtswg freq1 freq2 ... ...freqn -[q|e|b]
usage and options are the same as for swg (SineWaveGenerator).
-
rtfbp is the C++ source code for FloatBufferPlayer using RtAudio:
USAGE:
rtfbp sample_rate file_name <device_number> <channel_offset>
device_number and channel_offset values are optional.
-
rttfp is the C++ source code for TextFilePlayer using RtAudio:
USAGE:
rttfp sample_rate file_name <device_number> <channel_offset>
device_number and channel_offset values are optional.
-
rtpt is a slightly more complex demo program based on combining
concepts of
rtswg and Thread Demo
with source code adapted for synthesizing a large number of
partials, using a faster wavetable algoritm, parallel multi-processing,
TPCircularBuffer and
RtAudio:
USAGE:
rtpt num_partials fundamental_freq -[q|e|b]
options are the same as for rtswg (SineWaveGenerator).
-
rtpm is the summarizing demo program combinging the technologies of
wavetable algoritm oscillator bank synthesis, parallel multi-processing, POSIX threads,
circular buffering,
RtAudio and
RtMidi in the form of a
no GUI monophonic synthesizer.
USAGE:
rtpm num_partials -[q|e|b]
options are the same as for rtswg (SineWaveGenerator).
-
rtfbp1 is a version of rtfbp
written for connecting with Slightly more elaborate examles above:
USAGE:
rtfbp1 sample_rate file_name <device_number> <channel_offset>
device_number and channel_offset values are optional.
0 file_name tells program to pipe input data from another program:
EXAMPLE: com 6 | siner1 -db 0.2 | rtfbp1 44100 0
-
rttfp1 is a version of rttfp
written for connecting with Slightly more elaborate examles above:
USAGE:
rttfp1 sample_rate file_name <device_number> <channel_offset>
device_number and channel_offset values are optional.
0 file_name tells program to pipe input data from another program:
EXAMPLE: com 6 | siner1 -d 0.2 | rttfp1 44100 0
Utilities work with MacOS X 10.6 and 10.7, 10.8 and 10.10 but:
NO WARRANTY
download
starters ( -source only).
download
PartialsCatenator (MacOS X -binary / source).
download
Partials' Converters (MacOS X -binary / source).
download
PartialsTrackingFileResampler (source and MacOS X binary).
download
SineWaveGenerator (MacOS X -binary only).
download
FloatBufferPlayer (MacOS X -binary only).
download
TextFilePlayer (source and MacOS X binary).
download
FloatByteSwapper (MacOS X binary / source).
download
Thread Demo (MacOS X binary / source).
download
pri (source code).
download
com (source code).
download
genetic (source code).
download
siner1 (source code).
download
FloatBufferPlayer1 (source and MacOS X binary).
download
TextFilePlayer1 (source and MacOS X binary).
download
rtsine ( -source only ).
download
rtswg ( -source only ).
download
rtfbp ( -source only ).
download
rttfp ( -source only ).
download
rtpt ( -source only ).
download
rtm ( -source only ).
download
rtfbp1 ( -source only).
download
rttfp1 ( -source only).
and here's
the GUI FloatBufferPlayer (MacOS X -binary only).
I'll post all the source code soon, together with a small tutorial in writing such simplest audio
utilities.